Bite-Sized Reads
Short and sweet reads that take less than 10 minutes of your busy day!
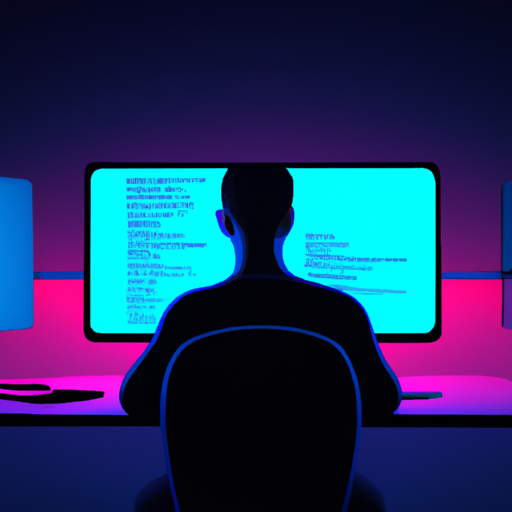
Title: "Why Writing Tests is Crucial for Fullstack Web Development with Laravel"
Writing tests in fullstack web development with Laravel is extremely important.
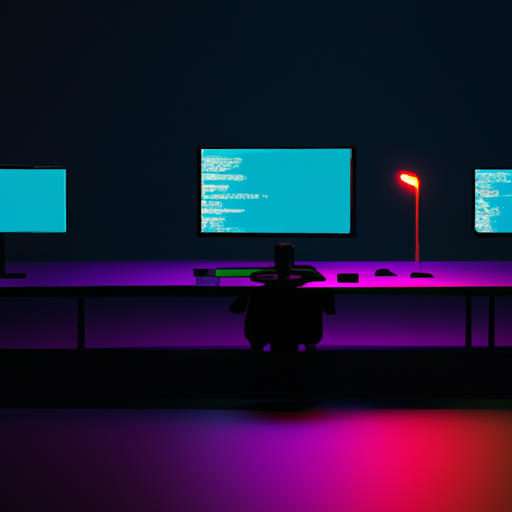
Title: "Building a Fullstack Web Application with Laravel and Vue.js"
In this tutorial, we will learn how to build a fullstack web application using Laravel and Vue.js.
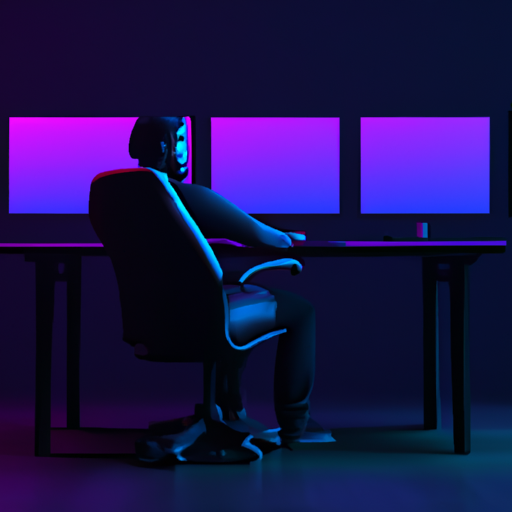
Building a RESTful API with Laravel: A Step-by-Step Guide for Fullstack Web Developers"
In this step-by-step guide, fullstack web developers can learn how to build a RESTful API with Laravel. The guide provides a comprehensive tutorial to help developers understand the process of creating a Laravel-based API.
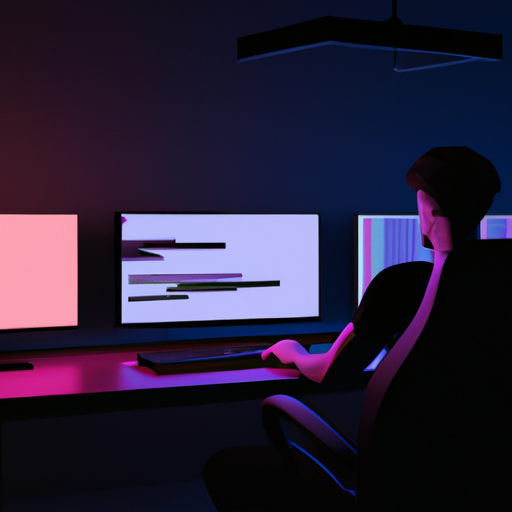
Title: "Why Writing Tests is Crucial in Fullstack Web Development"
Writing tests is an essential part of full-stack web development. With proper testing, developers can ensure that their code is performing as expected, catch and fix issues early, and prevent bugs from making it into production. This ultimately leads to higher quality software and a better user experience.
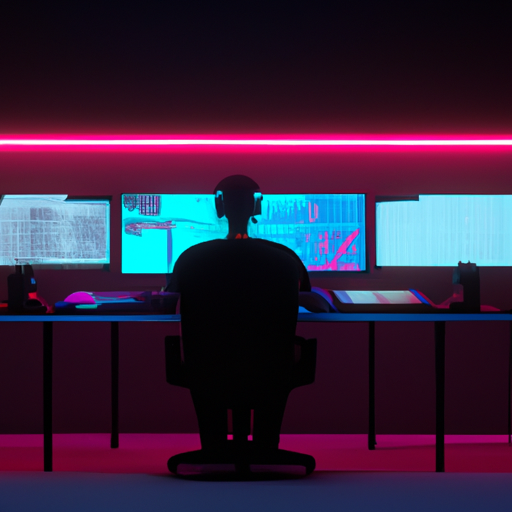
Title: "The Power of AI in Fullstack Web Development"
This document will focus on the benefits and power of using AI in fullstack web development. It will explore the ways in which AI can enhance the development process, improve user experience, and optimize website performance.
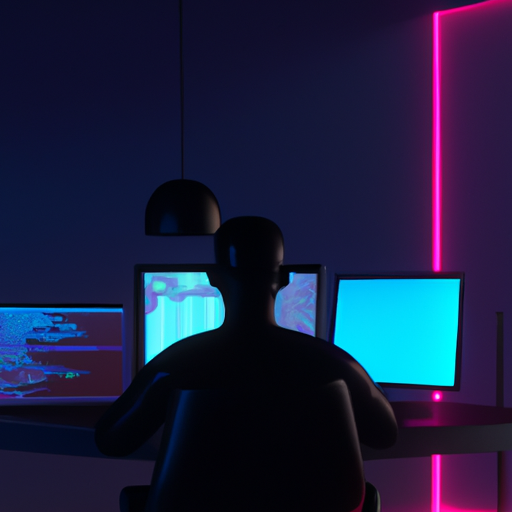
Title: "Building Robust REST APIs with Laravel: Tips and Best Practices"
In this article, we will explore tips and best practices for building robust REST APIs with Laravel - a popular PHP web framework.
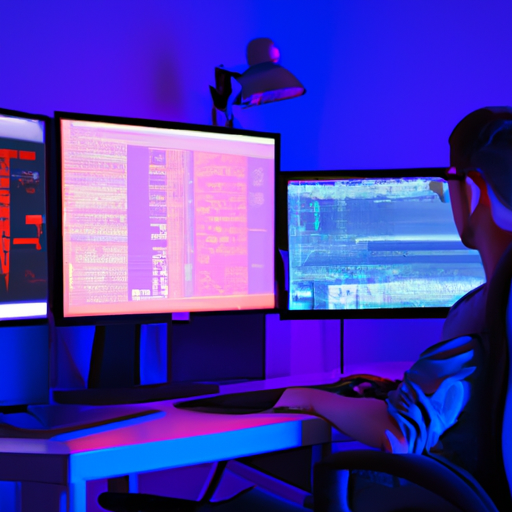
How to properly create route groups in laravel
To create route groups in Laravel, you can use the `Route::group()` method. This method allows you to group routes under a common prefix, middleware, and namespace. Here's an example of how to create a route group in Laravel: ```php Route::group(['prefix' => 'admin', 'middleware' => 'auth', 'namespace' => 'Admin'], function () { Route::get('/', 'DashboardController@index'); Route::resource('/users', 'UserController'); }); ``` In this example, we've created a route group with the prefix `admin`, which means that all routes within this group will have the `/admin` URL prefix. We've also specified that the `auth` middleware should be applied to all routes in this group, which means that only authenticated users will be able to access them. Finally, we've set the namespace to `Admin`, which means that all controller references within this group will be assumed to be in the `App\Http\Controllers\Admin
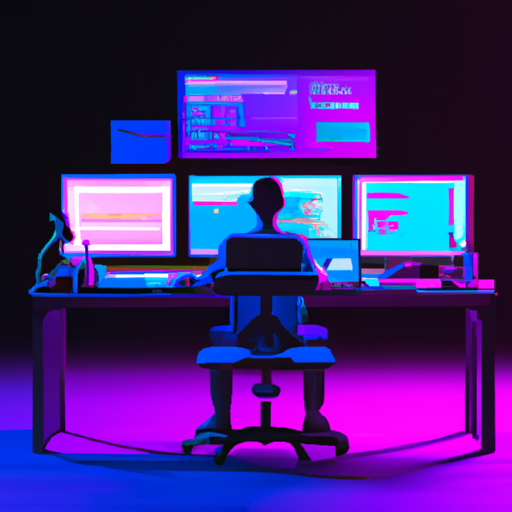
Mastering Test-Driven Development with Pest
In this article, we’ll look at how to get started with Test-Driven Development using the PHP testing framework called Pest. Test-driven development (TDD) is an essential aspect of software development, providing the confidence and peace of mind to continuously make improvements and updates to your application.
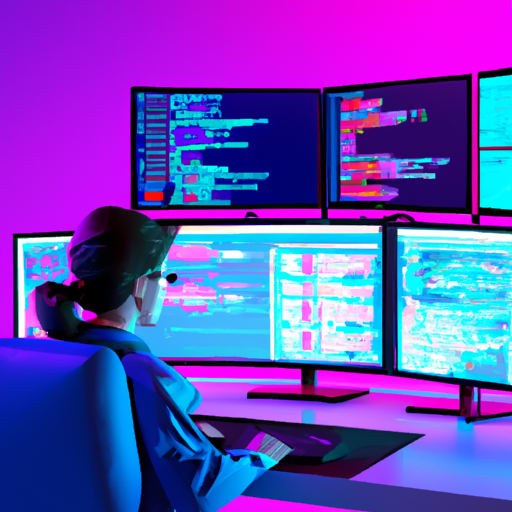
Build your first Laravel package
To create your first Laravel package, follow the steps, these involve: 1. Create a new Laravel app using Composer. 2. Create a new directory called `src` inside the package directory. 3. Create a new file called `ExamplePackageServiceProvider.php` inside the `src` directory. 4. In order for Laravel to detect the package's service provider, you need to add it to the Kernel. Your package should also have its Composer.json file where you can specify classes autoloading, dependecies and package details
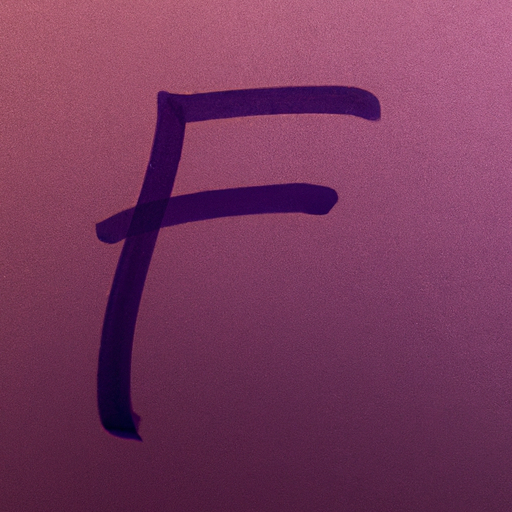
Use fetch to build better user experience
Suppose we have an input field for the user to enter the title of a blog post, and we want to generate a summary of the post based on the title. We can do this without the user having to refresh the page by using fetch
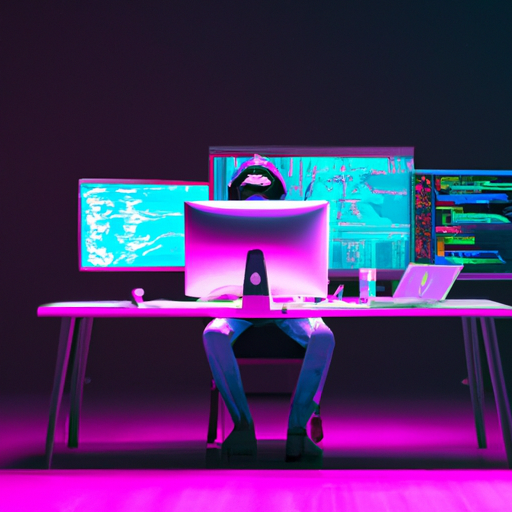
Vuejs tips and tricks, use the next-tick feature
Next-tick is a feature in Vue.js that allows developers to defer the execution of a function until the next tick of the JavaScript event loop. This is a useful feature when it comes to updating the view after a data change has occurred. The next-tick feature ensures that the DOM is updated only once after all the data changes have been processed, and this way, it reduces the number of updates and improves performance. In practice, this feature can be used to update the view after data changes have been processed in the application. For example, if a user types into an input field, the data will change, and the view will need to be updated. By using the next-tick feature, the view will be updated only once after all the data changes have been processed, which reduces the amount of work that needs to be done and improves the application's overall performance. Overall, Vue.js's next-tick feature is a useful tool that helps developers optimize their applications and create better user experiences.
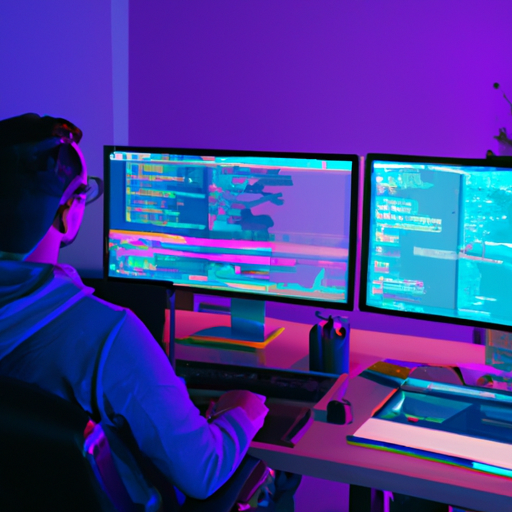
Laravel tips and tricks, how to define a custom middleware
Laravel middleware is a very powerful feature of the Laravel framework that can be used to filter HTTP requests passing through your application. In simple terms, middleware are functions that sit between the request and response of an application. The middleware can modify the request or response before it is sent to or received from the server. Laravel middleware can be used to secure routes, check if a user is authenticated, log requests, and many more. In summary, middleware is a feature that allows developers to filter and modify HTTP requests in Laravel web applications. Laravel middleware is easy to use and very flexible, allowing developers to implement custom middleware to meet their specific needs. With middleware in Laravel, developers can secure their applications, log requests, and perform a host of other functions to enhance the functionality and security of their web applications.